티스토리 뷰
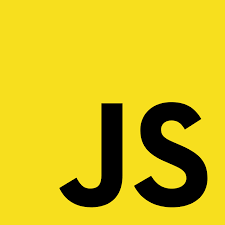
자바스크립트 언어의 특징상 null을 undefined로 표현합니다. 그리고 자바스크립트는 자바 언어와는 달리 인터프리터 방식의 언어이기 때문에 빌드를 할 때 에러가 나지도 않습니다. (물론 자바에서도 null 값이 들어갈 때 에러가 안 나고 있다가 런타임 에러가 발생하면 NullPointerException 이 발생합니다.) 그럼 이렇게 표현될 때 최대한 방어를 할 수 있는 Java의 Optional 클래스와 같이 쓸 수 있는 방법이 있을까요?
1. 기본 파라미터 값 넣기
함수(function)를 호출하는 파라미터에서 기본값을 설정할 수 있습니다.
function nullSafeMultiple(number = 1) {
return number * 2;
}
let num = undefined;
nullSafeMultiple(num); // 2;
위의 상황에서는 number == undefined 이기 때문에 number 의 기본값을 1로 변환해서 곱해주게 되고, 결과가 2가 나오게 됩니다.
2. Double negation 사용하기
이 문법은 이전부터 존재한 논리 연산자의 한 종류로 '!!'를 활용하여 null or undefined 값을 true, false로 변환해 주는 역할을 합니다.
class People {
constructor(name, age, height, company) {
this.name = name;
this.age = age;
this.height = height;
this.company = company;
}
}
class Company {
constructor(name, location) {
this.name = name;
this.location = location;
}
}
const people = new People(
age : 30, height : null, name : 'niyong'
)
const myHeight = !!people.height; // false
위의 경우 People 객체에서 키(height) 를 정해주지 않았고, people.height 가 원래의 케이스로는 null 이 나와야 합니다. 하지만!!로 wrapping 해주었기 때문에 존재하지 않는 값에 대해 false를 반환해 줍니다.
3. Optional Chaining
옵셔널 체이닝은 메소드의 내용에서 물음표 기호(?)를 붙여 해당 객체가 null 인 경우 undefined 를 리턴해주는 방법입니다.
class People {
constructor(name, age, height, company) {
this.name = name;
this.age = age;
this.height = height;
this.company = company;
}
}
class Company {
constructor(name, location) {
this.name = name;
this.location = location;
}
}
const people = new People(
age : 30, height : null, name : 'niyong', company : null
)
const myCompany = people.company?.location; // undefined
4. Nullish coalescing operator
이 방법은 물음표를 두 개 연속(??)으로 붙여서 사용하는 방법으로 변수 선언형 다음에 붙게 되어 기본값을 할당해 주는 방법입니다. 사용 방법은 아래와 같습니다.
class People {
constructor(name, age, height, company) {
this.name = name;
this.age = age;
this.height = height;
this.company = company;
}
}
class Company {
constructor(name, location) {
this.name = name;
this.location = location;
}
}
const people = new People(
age : 30, height : null, name : 'niyong', company : null
)
const myCompany = people.company ?? { name : 'abbo', location : 'Seoul' }
console.log(myCompany) // { name : 'abbo', location : 'Seoul' }
결론
이렇게 자바스크립트에서 변수의 값이 set되지 않을 때 반환하는 값을 포장할 수 있는 방법에 대해 알아보았습니다. Javascript의 특징상 값이 설정된 것을 일일이 jest와 같이 테스트 클래스를 설정하여 적용하는 방법이 생각보다 어렵기 때문에 변수의 값을 보호하는 차원 + Undefined error 아래에 선언된 javascript 를 호출할 때 방어하는 차원에서 유리하게 작용할 것입니다.
'Client' 카테고리의 다른 글
[Javascript] Spinner 구현하기 (1) | 2023.04.28 |
---|---|
[Web] View Transitions API 가 나오면서 화면 전환이 더 쉬워진다 (1) | 2023.04.02 |
[Javascript] 얕은 복사(Shallow Copy)와 깊은 복사(Deep Copy) (0) | 2023.03.26 |
[Flutter] Android Studio Live Template 설정하기 (0) | 2023.03.05 |
[Flutter] Android Studio SDK 설치 방법 및 호환되는 SDK 체크 (2) | 2023.03.04 |
[Flutter] 개요 및 환경 세팅하기 (0) | 2023.03.04 |